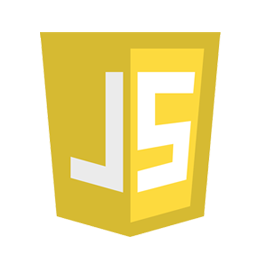
Object Prototypes
التاريخ
الدروس
المستوى
اللغة
المشاهدات
المواضيع
الشروحات chevron_left Object Prototypes chevron_left JavaScript
Object Prototypes
</> Object Prototypes
في الدروس السابقة تعلمنا كيفية استخدام ال object constructor
function Person(first, last, age, eyecolor) { this.firstName = first; this.lastName = last; this.age = age; this.eyeColor = eyecolor; } const myFather = new Person("ahmed", "hatem", 50, "blue"); const myMother = new Person("salah", "saleh", 48, "green");
تعلمنا أيضا انه لا نستطيع اضافة خاصية property جديدة لل constructor الخاص ب Object معين بالطريقة العادية التي نستخدمها لذلك
Person.nationality = "English";
وعرفنا انه يمكننا اضافة خاصية property جديدة ل object معين عن طريق اضافة هذه الخاصية بداخل دالة constructor
function Person(first, last, age, eyecolor) { this.firstName = first; this.lastName = last; this.age = age; this.eyeColor = eyecolor; this.nationality = "English"; }
</> Prototype Inheritance
تقوم ال objects في لغة الجافاسكريب بوراثة الخصائص properties والدوال methods من نموذج معين تم انشائه مسبقا prototype
- عنصر Date يتم وراثته من "Date.prototype "
- عنصر ال Array يتم وراثته من " Array.prototype "
- عنصر ال Person يتم وراثته من "person.prototype "
العنصر التالي "Object.prototype " هو النموذج الاعلي الذي يتم وراثة العناصر الاخري منه مثل (Array , Person Date )
- في كثير من الاحيان نحتاج الي اضافة دوال methods وخصائص properties الي object معين خلال العمل علي مشروع ما وذلك يمكننا تحقيقه عن طريق Prototype كالاتي
</> Adding Properties
نستطيع اضافة خاصية property الي object معين عن طريق استخدام ال prototype كما في المثال التالي
Example
function Person(first, last, age, eyecolor) { this.firstName = first; this.lastName = last; this.age = age; this.eyeColor = eyecolor; } Person.prototype.nationality = "English";
</> Adding Methods
نستطيع اضافة دالة Method الي object معين عن طريق استخدام ال prototype كما في المثال التالي
Example
function Person(first, last, age, eyecolor) { this.firstName = first; this.lastName = last; this.age = age; this.eyeColor = eyecolor; } Person.prototype.name = function() { return this.firstName + " " + this.lastName; };
Example
function Person(first, last, age, eyecolor) { this.firstName = first; this.lastName = last; this.age = age; this.eyeColor = eyecolor; } Person.prototype.nationality = "Arabic ";